Linting TypeScript with ESLint
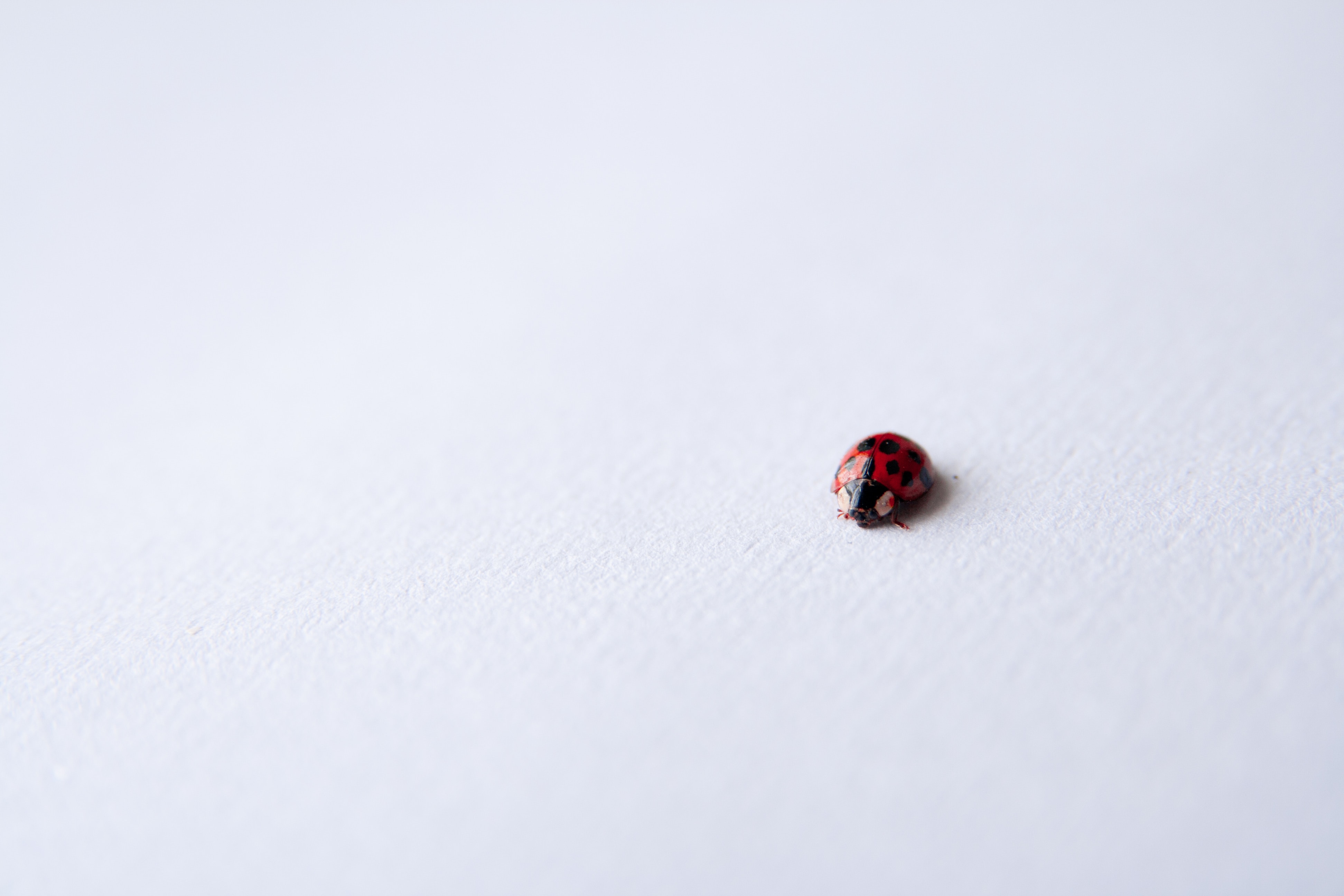
With Palantir announcing the deprecation of TSLint(blogpost), it's probably a good time to setup ESLint in your TypeScript project. You can also read more about the future of TypeScript on ESLint posted here.
Let's look at how we can add ESLint into a TypeScript project.
Setup Steps
$ yarn add -D eslint
$ yarn add -D @typescript-eslint/{eslint-plugin,parser}
-D
is short for--save-dev
yarn add @types/{name1,name2}
is short foryarn add @types/name1 @types/name2
Next, create a configuration file for ESLint. You'll need to add @typescript-eslint/parser
to the parser section and the "@typescript-eslint"
to the plugin section as below:
module.exports = {
"parser": "@typescript-eslint/parser",
"parserOptions": {
"sourceType": "module" // to support ImportDeclaration
},
"plugins": [
"@typescript-eslint",
]
}
Not sure which rules to add? You could start with the recommended rules and tweak the rules as you go to see which rules suits your project more. The recommended rules can be added as below:
+ "extends": [
+ "plugin:@typescript-eslint/recommended",
+ ],
With the above setup, the minimum configuration to add ESLint to a TypeScript project is done.
Other Helpful Configurations
Using ReactJS?
If you are using ReactJS in your project, you should add ReactJS specific ESLint rules available from eslint-plugin-react.
$ yarn add -D eslint-plugin-react
There is also a set of recommended rules for ReactJS that you could use by adding plugin:react/recommended
to the extends section below.
"extends": [
+ "plugin:react/recommended",
"plugin:@typescript-eslint/recommended",
],
Using Prettier?
If you're using Prettier, it is likely that the settings between prettier and ESLint indent contradicts each other. To fix that, you'll need to tell ESLint to use prettier instead and avoid seeing the @typescript-eslint/indent
errors.
$ yarn add -D eslint-config-prettier
"extends": [
"plugin:@typescript-eslint/recommended",
+ "prettier/@typescript-eslint",
],
Recommended prettier rules? Yes! Let's add it to the project.
$ yarn add -D eslint-plugin-prettier
"extends": [
"plugin:@typescript-eslint/recommended",
"prettier/@typescript-eslint",
+ "plugin:prettier/recommended"
],
Below is the overall minimum .eslintrc.js
configuration added in this post.
module.exports = {
"parser": "@typescript-eslint/parser",
"parserOptions": {
"sourceType": "module"
},
"plugins": [
"@typescript-eslint",
],
"extends": [
"plugin:react/recommended",
"plugin:@typescript-eslint/recommended",
"prettier/@typescript-eslint",
"plugin:prettier/recommended"
],
}
Using vscode?
Adding ESLint extension would greatly help in your development experience. Then, proceed to add the configuration below to your settings.json to include validation for typescript and typescriptreact(if you are using ReactJS).
"eslint.validate": [
{ "language": "typescript" },
{ "language": "typescriptreact" }
]
Additionally, if you want to include autofixing of the lint rules on file save. Autofixing would only work with lint rules that are auto fixable with the --fix
flag.
"eslint.autoFixOnSave": true,
"eslint.validate": [
{ "language": "typescript", "autoFix": true },
{ "language": "typescriptreact", "autoFix": true }
]
Additional Information
Check this helpful library called tslint-to-eslint-config that converts the TSLint configuration to the closest possible ESLint configuration.
📝 Do make sure to upgrade your TSLint to 5.18.0 as the added --print-config
CLI flag is needed for the library above.
Happy linting~!
Clap to support the author, help others find it, and make your opinion count.