Functions & Lambdas in Kotlin
While coding at work today, I discover a few ways for referencing methods in Kotlin.
In Kotlin, we can declare and pass lambda like any normal type objects into methods. Then they can be executed by calling methodName.invoke()
or directly using methodName()
:
Let's say we have a function like this:
helloWorldFunction.kt
fun helloWorldFunction(year: Int): String {
return "[function] year ${year}"
}
it can be called like this:
main.kt
helloWorldFunction(2018)
// output: [function] year 2018
We can also assign it into a variable, then call it
Note that we need ::
to reference to a method name.
main.kt
val target = ::helloWorldFunction
println(target(2018))
// output: [function] year 2018
Instead of a function, we can also create a lambda:
helloWorldLambda.kt
var helloWorldLambda = { year: Int ->
"[lambda] year ${year}"
}
Call side would look like this:
main.kt
val target = helloWorldLambda
println(target(123))
// output: [lambda] year 2018
There is also an alternative way of calling the function
or lambda
from the assigned variable
:
both_works.kt
target(123)
target.invoke(123)
scoped methods
There is another interesting thing that I learned about Kotlin's function and lambda. Scope can be applied to them.
scoped_function.kt
fun String.surroundWithNumber(number: Int): String {
return "${number}_${this}_${number}"
}
The prefix in front of the method name, String.
, means that it should be called by a String
object. "randomString".surroundWithNumber(0)
works fine, but surroundWithNumber(0)
will give you a syntax error.
Again, this can be assigned into a variable, and get passed around and executed:
main.kt
var target: String.(Int) -> String
target = String::surroundWithNumber
println("random".target(999))
// output: 999_random_999
Pretty cool features
See you in the next post!
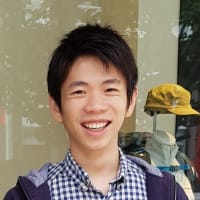
Tan Jun Rong
Clap to support the author, help others find it, and make your opinion count.